반응형
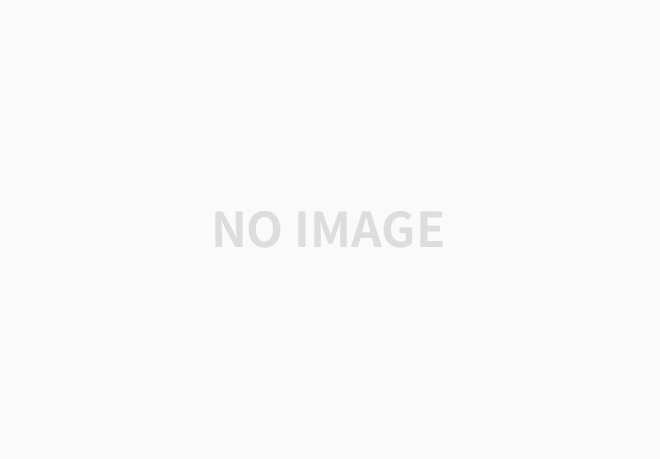
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#region Question 3.7 | |
/* | |
* 먼저 들어온 동물이 먼저 나가는 동물쉼터가 있다고 하자. 쉼터는 개와 고양이만 수용할 수 있다. | |
* 사람들은 쉼터의 동물들 가운데 들어온 지 가장 오래된 동물부터 입양할 수 있는데, 개와 고양이 중, | |
* 어떤 동물을 데려갈지 선택할 수도 있다. 특정한 동물을 지정해 데려가는 것은 금지되어 있다. | |
* (enqueue, dequeueAny, dequeueDog, dequeueCat의 연산을 제공해야 한다.) | |
*/ | |
public class AnimalShelter | |
{ | |
public abstract class Animal : IComparable<Animal> | |
{ | |
public DateTime Time { get; } | |
public string Name { get; } | |
protected Animal(string name, DateTime time) | |
{ | |
this.Name = name; | |
this.Time = time; | |
} | |
public int CompareTo(Animal other) | |
{ | |
return this.Time.CompareTo(other.Time); | |
} | |
#region Method Overloading | |
public static bool operator >(Animal b1, Animal b2) | |
{ | |
return (b1.CompareTo(b2) > 0); | |
} | |
public static bool operator <(Animal b1, Animal b2) | |
{ | |
return (b1.CompareTo(b2) < 0); | |
} | |
#endregion | |
} | |
public class Cat : Animal | |
{ | |
public Cat(string name, DateTime time) : base(name, time) | |
{ | |
} | |
} | |
public class Dog : Animal | |
{ | |
public Dog(string name, DateTime time) : base(name, time) | |
{ | |
} | |
} | |
private Queue<Dog> dogs; | |
private Queue<Cat> cats; | |
public AnimalShelter() | |
{ | |
this.dogs = new Queue<Dog>(); | |
this.cats = new Queue<Cat>(); | |
} | |
public void Enqueue(Animal animal) | |
{ | |
switch (animal) | |
{ | |
case Dog d: | |
this.dogs.Enqueue(animal as Dog); | |
break; | |
case Cat c: | |
this.cats.Enqueue(animal as Cat); | |
break; | |
} | |
} | |
public Animal DequeueAny() | |
{ | |
if(dogs.Count == 0) | |
{ | |
return this.DequeueCat(); | |
} | |
if(cats.Count == 0) | |
{ | |
return this.DequeueDog(); | |
} | |
if(dogs.Peek() < cats.Peek()) | |
{ | |
return this.DequeueDog(); | |
} | |
else | |
{ | |
return this.DequeueCat(); | |
} | |
} | |
public Animal DequeueCat() | |
{ | |
try | |
{ | |
return this.cats.Dequeue(); | |
} | |
catch (InvalidOperationException) | |
{ | |
return null; | |
} | |
} | |
public Animal DequeueDog() | |
{ | |
try | |
{ | |
return this.dogs.Dequeue(); | |
} | |
catch (InvalidOperationException) | |
{ | |
return null; | |
} | |
} | |
} | |
#endregion |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
[TestMethod] | |
public void Q3_7() | |
{ | |
var shelter = new StackQueue.AnimalShelter(); | |
shelter.Enqueue(new StackQueue.AnimalShelter.Cat("A", new DateTime(100))); | |
Assert.IsNull(shelter.DequeueDog()); | |
Assert.AreEqual("A", shelter.DequeueCat().Name); | |
shelter.Enqueue(new StackQueue.AnimalShelter.Cat("B", new DateTime(200))); | |
Assert.AreEqual("B", shelter.DequeueAny().Name); | |
shelter.Enqueue(new StackQueue.AnimalShelter.Dog("C", new DateTime(300))); | |
shelter.Enqueue(new StackQueue.AnimalShelter.Cat("D", new DateTime(400))); | |
Assert.AreEqual("C", shelter.DequeueAny().Name); | |
} |
반응형
'Develop > 코딩인터뷰' 카테고리의 다른 글
[코딩인터뷰] 문제 8.1 (0) | 2020.04.04 |
---|---|
[코딩인터뷰] 문제 8.7 (0) | 2020.04.04 |
[코딩인터뷰] 문제 3.6 (0) | 2020.04.03 |
[코딩인터뷰] 문제 3.5 (0) | 2020.04.03 |
[코딩인터뷰] 문제 3.4 (0) | 2020.04.03 |
꾸준히 노력하는 개발자 "김예건" 입니다.