반응형
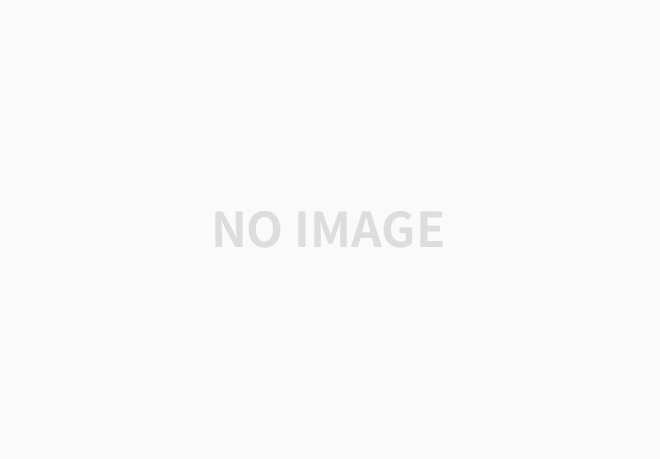
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#region Question 3.5 | |
/* | |
* 두 개의 스택을 사용하여 큐를 구현하는 MyQueue 클래스를 작성해보라. | |
*/ | |
/// <summary> | |
/// 두 개의 스택으로 구현한 큐 자료구조 | |
/// </summary> | |
/// <typeparam name="T">저장할 데이터 타입</typeparam> | |
public class MyQueue<T> | |
{ | |
private readonly Stack<T> stackEnqueue; | |
private readonly Stack<T> stackDequeue; | |
public MyQueue() | |
{ | |
stackEnqueue = new Stack<T>(); | |
stackDequeue = new Stack<T>(); | |
} | |
public int Size() | |
{ | |
return stackEnqueue.Count + stackDequeue.Count; | |
} | |
public void Enqueue(T value) | |
{ | |
stackEnqueue.Push(value); | |
} | |
private void SwitchStack() | |
{ | |
// 꺼낼 스택이 비어있을 때만 스택 데이터를 옮긴다. | |
if (stackDequeue.Count == 0) | |
{ | |
while (stackEnqueue.Count != 0) | |
{ | |
stackDequeue.Push(stackEnqueue.Pop()); | |
} | |
} | |
} | |
public T Peek() | |
{ | |
SwitchStack(); | |
return stackDequeue.Peek(); | |
} | |
public T Dequeue() | |
{ | |
SwitchStack(); | |
return stackDequeue.Pop(); | |
} | |
} | |
#endregion |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
[TestMethod] | |
public void Q3_5() | |
{ | |
var myQueue = new StackQueue.MyQueue<int>(); | |
myQueue.Enqueue(1); | |
myQueue.Enqueue(2); | |
Assert.AreEqual(1, myQueue.Peek()); | |
Assert.AreEqual(1, myQueue.Dequeue()); | |
myQueue.Enqueue(3); | |
Assert.AreEqual(2, myQueue.Dequeue()); | |
Assert.AreEqual(3, myQueue.Peek()); | |
myQueue.Enqueue(4); | |
Assert.AreEqual(3, myQueue.Peek()); | |
Assert.AreEqual(3, myQueue.Dequeue()); | |
Assert.AreEqual(4, myQueue.Dequeue()); | |
} |
반응형
'Develop > 코딩인터뷰' 카테고리의 다른 글
[코딩인터뷰] 문제 3.7 (0) | 2020.04.03 |
---|---|
[코딩인터뷰] 문제 3.6 (0) | 2020.04.03 |
[코딩인터뷰] 문제 3.4 (0) | 2020.04.03 |
[코딩인터뷰] 문제 3.3 (0) | 2020.04.03 |
[코딩인터뷰] 문제 3.2 (0) | 2020.04.03 |
꾸준히 노력하는 개발자 "김예건" 입니다.